Tuesday, February 10, 2015
Java Interview Questions
Here I am providing the most important java interview questions that are frequently asked in interviews. If you want to submit any core java or advance java interview questions or if you find any mistake in below questions than please notify us by the contact us page.
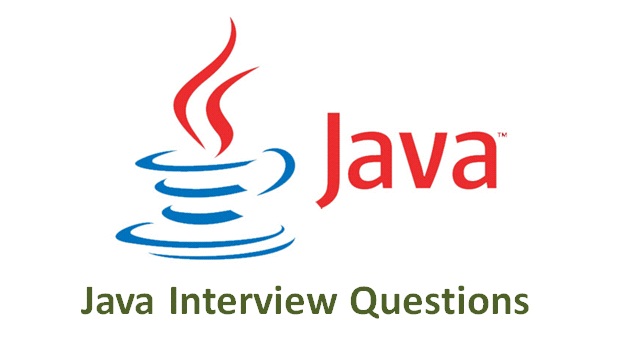
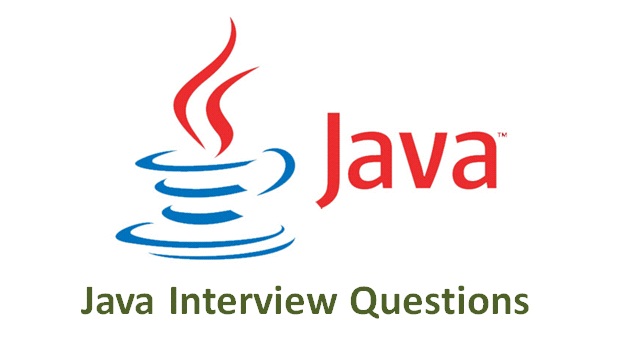
Core Java Interview Questions
Q. Can we keep more than one class in a single java file?
A. Yes
Q. Can we keep main method in each class?
A. Yes
Q. Can we keep more than one main method in a single class?
A. Yes, with different prototype.
class A
{
public static void main(String...s)
{
System.out.println("Original main method");
}
public static void main(int s1)
{
System.out.println("Duplicate main method");
}
}
Q. Can we call main method explicitly?
A. Yes
class A
{
public static void main(String...s)
{
System.out.println("The Crazy Programmer");
}
}
class B
{
public static void main(String...s)
{
A.main();
}
}
Q. Can we execute our program without main method?
A. Yes, by using static block (supported till jdk 1.6).
class A
{
static
{
System.out.println("The Crazy Programmer");
}
}
Q. Why character takes 2 bytes in java?
A. Because java support UNICODE, and for supporting UNICODE minimum 2 bytes are required.
Q. Is java purely or truly object oriented language?
A. No, because it supports primitive data types.
Q. Does constructor return any value?
A. Yes, it returns current object reference (this).
Q. Constructor is called before or after object creation?
A. It is called at the time of creation of object.
Q. Constructor is static or non-static?
A. 90% constructor is static by nature.
Q. Can constructor overloaded?
A. Yes, constructor is special method and method can be overloaded.
Q. What is the use of default constructor?
A. To initialize the default value.
Q. Inheritance occurs at compile time or run time?
A. At runtime, it can be proved by simply decompiling the .class file of child class.
Q. What super holds?
A. It holds reference id of parent section of child class object.
Q. What is first line of constructor, this or super?
A. By default first line of constructor is super. Both super and this can’t be used together.
Q. Why we use dynamic binding?
A. To achieve abstraction.
Q. Can we keep constructor in abstract class?
A. Yes, each class in java have constructor.
Q. Can we make abstract method static?
A. No, because static is accessed via class name and it will be accessed by anyone.
Q. Why we make interface methods by default public and abstract?
A. Because methods are implemented by end user.
Q. How multiple inheritance is implemented in java?
A. Multiple inheritance is implemented by interface.
interface my1
{
. . . . .
. . . . .
}
interface my2
{
. . . . .
. . . . .
}
interface my3 extends m1,m2
{
. . . . .
. . . . .
}
Q. What is difference between String and StringBuffer?
A. In String object is immutable (can’t be modified) while in StringBuffer object is mutable (can be modified).
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.